One interviewer asked me a question, “Do you know about Java Memory Management “. I started replying like this, “Heap and Stack are the parts of it. Heap is the place where we store the objects and stack is the place for storing the reference for those objects.” He started firing subsequent questions and I was stunned,
- What are all the layers inside java memory ?
- What happens to the unused object ?
- What is garbage collector ?
- Is it good to run garbage collector often ?
- What is minor garbage and major garbage collector ?
In this article, I am going to understand java memory management and will find answers for above questions. Let’s dive in..
The Heap and the Nursery
- Java objects reside in an area called the heap.
- The heap is created when the JVM starts up and may increase or decrease in size while the application runs.
- When the heap becomes full, garbage is collected.
- During the garbage collection objects that are no longer used are cleared, thus making space for new objects.
The heap is divided into 2 areas (or generations)
- Nursery (or young space)
- Old space
Nursery is a part of heap reserved for the allocation of new objects. When the nursery becomes full , garbage is collected by running a special young collection, where all objects that have lived long enough in the nursery are promoted (moved) to the old space, thus freeing up the nursery for more object allocation. When the old space becomes full garbage is collected there, a process called an old collection.
In later java releases, a part of the nursery is reserved as a keep area. The keep area contains the most recently allocated objects in the nursery and is not garbage collected until the next young collection. This prevents objects from being promoted just because they were allocated right before a young collection started.
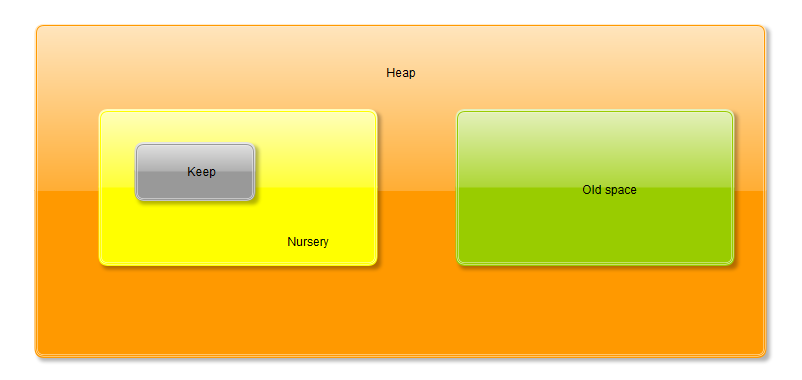
Object Allocation
During object allocation, the JRockit JVM distinguishes between small and large objects.
Small objects are allocated in thread local areas (TLAs). The thread local areas are free chunks reserved from the heap and given to a Java thread for exclusive use. The thread can then allocate objects in its TLA without synchronizing with other threads. When the TLA becomes full, the thread simply requests a new TLA. The TLAs are reserved from the nursery if such exists, otherwise they are reserved anywhere in the heap.
Large objects that don’t fit inside a TLA are allocated directly on the heap. When a nursery is used, the large objects are allocated directly in old space. Allocation of large objects requires more synchronization between the Java threads, although the JRockit JVM uses a system of caches of free chunks of different sizes to reduce the need for synchronization and improve the allocation speed.
Let’s take a look at Garbage Collectors in next post..